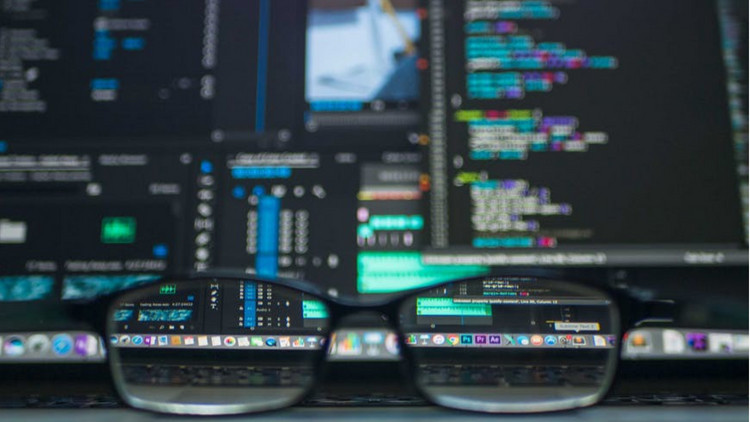
Course Information
Web development is the work involved in developing a web site for the Internet (World Wide Web) or an intranet (a private network). Web development can range from developing a simple single static page of plain text to complex web-based internet applications (web apps), electronic businesses, and social network services.
Following professionals can go for it:
- Fresher’s
- IT Experts
Eligibility: Technical graduate having fundamental knowledge of any programming languages like C, C++, Java, HTML. JavaScript will be benefited but not mandatory. We will provide a foundational and basics that are required.
Lecture Duration: 6 months
Placement: 100% Placement Assistance
Job Profile: Php developer, web developer
Php Professional Track
Content
PHP Introduction
- Install PHP
- PHP Echo
- PHP print
- Php Variable
- PHP constant
- PHP comments
- Control Statement
- PHP If else
- Php switch
- PHP For Loop
- PHP while loop
- PHP Do While Loop
- PHP Break
- PHP Functions
PHP Array
- PHP Indexed Array
- PHP Multidimenssional Array
- PHP Associatve Array
- PHP Array Functions
PHP Strings
- PHP Strings
- PHP String Function
- PHP Math Function
- PHP Form Handlling
- PHP Include and require
PHP Files
- PHP Open File
- PHP Read File
- PHP Write File
- PHP Append File
- PHP Delete File
PHP MYSQLI
- MYSQLI Connect
- MYSQLI Create DB
- MYSQLI Create Table
- MYSQLI Insert
- MYSQLI Update
- MySQLI Delete
- MYSQLI Select
Web Development
HTML & CSS Overview
Introduction
- HTML Basics
- HTML Elements
- HTML Attributes
- HTML Styles
- HTML Forms
- HTML Form Elements
- HTML Input Element Types
- HTML Input Attributes
- HTML File Paths
- Script tag and its uses
- HTML & XHTML
- CSS Introduction
- CSS Syntax
- CSS Selectors
- CSS Styling
Javascript Primer
- Introduction to Javascript
- Javascript Statements
- Javascript Keywords
- Javascript Functions
- Javascript Programs
- Javascript Operators
- Function Parameters
- Function Return Values
- Javascript Data Types
- Primitive Types
Working with Objects
- Object Overview
- Object Oriented Programming
- Object creation
- Adding Properties to Objects
- Adding Methods to Objects
- Javascript Conditional Statements
- Javascript Loops & Iteration
- Enumerating properties
- Callbacks
- JSON
- Environmental setup
MVC Architecture
- Model-View-Controller explained
- Why MVC matters
- MVC – the AngularJS way
First Application
Directives
- Introduction to Directives
- Directive lifecycle
- Using AngularJS built-in directives
- Binding controls to data
- Matching directives
- Creating a custom directive
Expressions
- Controllers
- Role of a Controller
- Attaching properties and functions to scope
- Nested Controllers
- Using filters in Controllers
- Controllers in External Files
- Controllers & Modules
Filters
- Built-in filters
- Using AngularJS filters
- Creating custom filters
- Tables
HTML DOM
- Modules
- Introduction to AngularJS Modules
- Bootstrapping
Forms
- Working with Angular Forms
- Model binding
- Form controller
- Validating Angular Forms
- Form events
- Updating models with a twist
- $error object
Scope
- What is scope
- Scope lifecycle
- Two way data binding
- Scope inheritance
- Scope & controllers
- Scope & directives
- $apply and $watch
- Rootscope
- Scope broadcasting
Dependency Injection & Services
- What is Dependency Injection
- Using Dependency Injection
- What are services
- Creating services
- Factory, Service & Provider
- Using AngularJS built in services
Single Page Application(SPA)
- What is SPA
- Pros & Cons of SPA
- Installing the ngRoute module
- Configure routes
- Passing parameters
- Changing location
- Resolving promises
- Create a Single Page Application
Angular-5
Getting Started
- Course Introduction.
- What is Angular?
- Angular vs Angular 2 vs Angular 4+
- Project Setup and First App.
- Editing the First App.
- The Course Structure.
- What is TypeScript.
- A Basic Project Setup using Bootstrap for Styling
The Basics
How an Angular App gets Loaded and Started Components
Components
Using Custom Components
Creating Components with the CLI & Nesting Components
Working with Component Templates
Working with Component Style
Assignment 1: Practicing Components
What is Databinding
- String Interpolation
- Property Binding
- Property Binding vs String Interpolation
- Event Binding
- Bindable Properties and Events
- Passing and Using Data with Event Binding
- Two-Way-Databinding
- Important: FormsModule is Required for Two-Way-Binding
- Combining all Forms of Databinding
Assignment 2: Practicing Databinding
- Understanding Directives
- Using ngIf to Output Data Conditionally
- Enhancing ngIf with an Else Condition.
Components & Databinding Deep Dive
- Splitting Apps into Components
- Property & Event Binding Overview
- Binding to Custom Properties
- Assigning an Alias to Custom Properties
- Binding to Custom Events
- Assigning an Alias to Custom Events
- Custom Property and Event Binding Summary
- Understanding View Encapsulation
- More on View Encapsulation
- Using Local References in Templates.
- Getting Access to the Template & DOM with @ViewChild.
- Understanding the Component Lifecycle
Lifecycle Hooks
Assignment 3: Practicing Property & Event Binding and View Encapsulation
Directives Deep Dive
- ngFor and ngIf ngClass and ngStyle
- How to create a Basic Directive
- Using Services & Dependency Injection Why would you Need Services?
- Creating a Logging Service
- Injecting the Logging Service into Components Creating a Data Service
- Understanding the Hierarchical Injector
- How many Instances of Service Should It Be? Injecting Services into
- Services
- Using Services for Cross-Component Communication
Assignment 4: Practicing Services
Changing Pages with Routing
- Why do we need a Router?
- Understanding the Example Project.
- Setting up and Loading Routes.
- Navigating with Router Links.
- Understanding Navigation Paths.
- Styling Active Router Links.
Navigating Programmatically
- Using Relative Paths in Programmatic Navigation Passing Parameters to Routes
- Fetching Route Parameters
- Fetching Route Parameters Reactively
- An Important Note about Route Observables Passing Query Parameters and Fragments Retrieving Query Parameters and Fragments Setting up Child (Nested) Routes
- Using Query Parameters – Practice Configuring the Handling of Query Parameters Redirecting and Wildcard Routes
- Important: Redirection Path Matching An Introduction to Guards
- Protecting Routes with canActivate
- Protecting Child (Nested) Routes with canActivateChild Using a Fake Auth Service
Controlling Navigation with canDeactivate
- Handling Forms in Angular Apps
- Template-Driven (TD) vs Reactive Approach
- TD Forms
Assignment 5: Practicing Template-Driven Forms
- Introduction to the Reactive Approach
- Reactive Forms
Assignment 6: Practicing Reactive Forms
- Using Pipes to Transform Output
- Introduction & Why Pipes are Useful Using Pipes
- Making Http Requests
- Introduction & How Http Requests Work in SPAs
- Sending Requests (Example: POST Request)
- Adjusting Request Headers
- Sending GET Requests Sending a PUT Request
- Transform Responses Easily with Observable Operators (map())
- Using the Returned Data
- Catching Http Errors
- Using the “async” Pipe with Http Requests.
Authentication & Route Protection in Angular Apps
- How Authentication Works in Single-Page-Applications
- Creating a Signup Page and Route
- Setting up the Firebase SDK Signing Users Up
- Signin Users In
- Requiring a Token (on the Backend) Sending the Token
- Checking and Using Authentication Status
- Adding a Logout Button
- Route Protection and Redirection. Wrap Up
- The HttpClient (ANGULAR 5 Addition Bonus SECTION)
- Request Configuration and Response.
- Requesting Events.
- Setting Headers.
- Interceptors.
Software Testing
Duration: 12 hrs.
Software Development Life Cycle :
- What are the different phases of SDLC?
- How does the process of Software Development Start?
- Project Initiation
Requirement Gathering and Analysis
- What is Requirement document and what it contains?
- What is use case document and what it contains?
- What is Basic path and Alternate Path?
- Role of Business Analyst
- Example for explaining each phase
- Role of technical specification team
- What is Technical specification document?
What is System Design?
- Role of Design team
- What is design document?
- Role of architecture team
System development
- Role of development team
- Deliverable of Development phase
System testing
- Role of testers and types of testing
- User acceptance testing
- System deployment
System maintenance
- Events in the maintenance phase like bug fixes
Software Testing Life Cycle
- How are the phases of STLC carried out?
- What is testing?
- Role of testers
- Why do we need to test?
- Activities involved in the testing phase
What is test plan and test case document?
- Steps of test case execution
- What does test case document contain?
- How to write test case document?
- What is required to test any application?
TEST CASES
- What is test case?
- What does test case document contain?
- How to write test case document?
- Different test case techniques
TEST PLAN
- What is Test Plan?
- How to write test plan document?
- What does the test plan document contain?
- Who writes and approves the test plan document?
- How manage the test case documents?
- What is the pass/fail criterion?
TYPES OF TESTING
- Different Phases of testing
- What is unit testing?
- What is Minimum acceptance testing?
- What is integration, system and system integration testing?
- What is User acceptance testing?
- What is Regression Testing?
DEFECT ANALYSIS
- What is a defect?
- Various Defect tracking tools
- How to use the defect tracking tools?
- How to enter the details of defect in the defect tracking tool?
- How to identify a defect?
- What is severity and priority?
TRACEABILITY MATRIX
- What is Traceability Matrix[TM]?
- Who Prepares the TM document?
- What is the reference for writing TM?
- What is the use of TM?
- What is present in the TM document?
- Sample TM
- Tools used for developing TM
Enquire Now !!!!! for online website development certification course and training in Kharghar, Navi Mumbai.